What?
Implementing claim-based authorization with tokens, refresh tokens, and MongoDB in an Express.js application requires several steps. Claim-based authorization allows you to grant or deny access to certain resources based on the claims contained within a user's token. Here's a high-level overview of how to implement this:
Set up your Express.js application:
Make sure you have Express.js installed and create a new Express.js project if you haven't already.
You'll need several packages to implement token-based authentication with MongoDB. Install them using npm or yarn:
npm install express mongoose jsonwebtoken bcrypt cors body-parser
Create an Express.js application and connect to MongoDB:
Set up your Express.js server and connect it to your MongoDB database. You can use the Mongoose library to interact with MongoDB.
const express = require('express');
const mongoose = require('mongoose');
const bodyParser = require('body-parser');
const cors = require('cors');
const app = express();
mongoose.connect('mongodb://localhost/your-database-name', { useNewUrlParser: true, useUnifiedTopology: true });
mongoose.connection.on('error', console.error.bind(console, 'MongoDB connection error:'));
app.use(bodyParser.urlencoded({ extended: false }));
app.use(bodyParser.json());
app.use(cors());
Define the user model:
Create a user model that represents the users in your application. The user model should have fields for username, password (hashed), and any claims (roles or permissions) associated with the user.
const mongoose = require('mongoose');
const Schema = mongoose.Schema;
const userSchema = new Schema({
username: String,
password: String, // Store hashed password
claims: [String], // An array of user claims (e.g., roles or permissions)
});
const User = mongoose.model('User', userSchema);
module.exports = User;
Implement user registration and authentication:
Create routes to register and authenticate users. When a user registers, you should hash their password and save their information to MongoDB. When a user logs in, verify their credentials, and if they are valid, create a JWT (JSON Web Token) with the user's claims and send it back to the client.
const express = require('express');
const jwt = require('jsonwebtoken');
const bcrypt = require('bcrypt');
const User = require('./models/user');
const router = express.Router();
router.post('/register', async (req, res) => {
const { username, password, claims } = req.body;
try {
const hashedPassword = await bcrypt.hash(password, 10);
const user = new User({ username, password: hashedPassword, claims });
await user.save();
res.status(201).send('User registered successfully');
} catch (error) {
res.status(500).send('Error registering user');
}
});
router.post('/login', async (req, res) => {
const { username, password } = req.body;
try {
const user = await User.findOne({ username });
if (!user) {
return res.status(401).send('Authentication failed');
}
const passwordMatch = await bcrypt.compare(password, user.password);
if (passwordMatch) {
const token = jwt.sign({ username, claims: user.claims }, 'your-secret-key', { expiresIn: '1h' });
res.status(200).json({ token });
} else {
res.status(401).send('Authentication failed');
}
} catch (error) {
res.status(500).send('Error during authentication');
}
});
module.exports = router;
Implement token verification middleware:
Create middleware to verify tokens before allowing access to protected routes.
const jwt = require('jsonwebtoken');
function verifyToken(req, res, next) {
const token = req.headers.authorization;
if (!token) {
return res.status(403).send('No token provided');
}
jwt.verify(token, 'your-secret-key', (err, decoded) => {
if (err) {
return res.status(401).send('Failed to authenticate token');
}
req.user = decoded;
next();
});
}
module.exports = verifyToken;
Protect routes with claim-based authorization:
Apply the verifyToken middleware to any routes you want to protect. Then, check the user's claims to determine if they have access to the requested resource.
const express = require('express');
const verifyToken = require('./verifyToken');
const router = express.Router();
router.get('/protected', verifyToken, (req, res) => {
// Check user claims to determine if they have access
const userClaims = req.user.claims;
if (userClaims.includes('admin')) {
res.status(200).send('You have access to this resource');
} else {
res.status(403).send('You do not have access to this resource');
}
});
module.exports = router;
Implement refresh tokens:
To implement refresh tokens, you can create an additional route that issues a new access token when the old one expires. This route should check the user's identity and claims and issue a new token with a new expiration time.
const express = require('express');
const jwt = require('jsonwebtoken');
const verifyToken = require('./verifyToken');
const router = express.Router();
router.post('/refresh-token', verifyToken, (req, res) => {
// Check user claims or any other criteria for issuing a new token
const userClaims = req.user.claims;
if (userClaims.includes('admin')) {
const token = jwt.sign({ username: req.user.username, claims: userClaims }, 'your-secret-key', { expiresIn: '1h' });
res.status(200).json({ token });
} else {
res.status(403).send('You do not have access to refresh your token');
}
});
module.exports = router;
Use these routes in your main Express.js app:
Finally, use the defined routes in your Express.js app:
app.use('/auth', require('./authRoutes'));
app.use('/api', require('./protectedRoutes'));
app.use('/auth', require('./refreshTokenRoutes'));
const PORT = process.env.PORT || 3000;
app.listen(PORT, () => {
console.log(`Server is running on port ${PORT}`);
});
Protect your secret key and use environment variables:
Make sure to protect your secret key and use environment variables to store sensitive information like the secret key, database connection string, and other configurations. This implementation is a basic example and should be adapted to your specific requirements and use case. You can expand on this foundation to include more advanced features like token revocation, user management, and role-based access control.
Demo
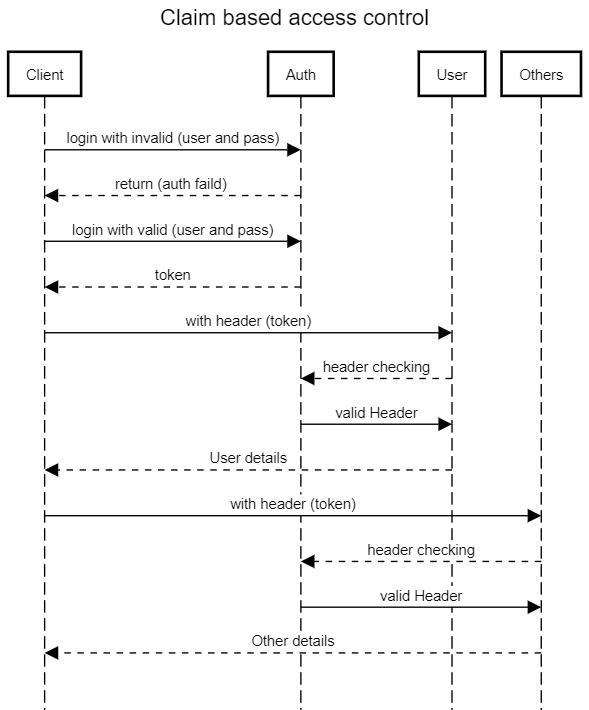